글이 많이 길기때문에 필요한 부분만 보도록 더보기를 이용했습니다.
클릭을 통해 필요한부분만 간편히 확인해주세요
0. 사용하는이유
더보기
JPA를 이용하는 것은 기존의 방식인 Connection, Statement, ResultSet 등을 불러오는 수고로움을 줄여준다.
그렇기때문에 이것을 사용하는법을 배운다.
1. 사용법
▶Controller
더보기
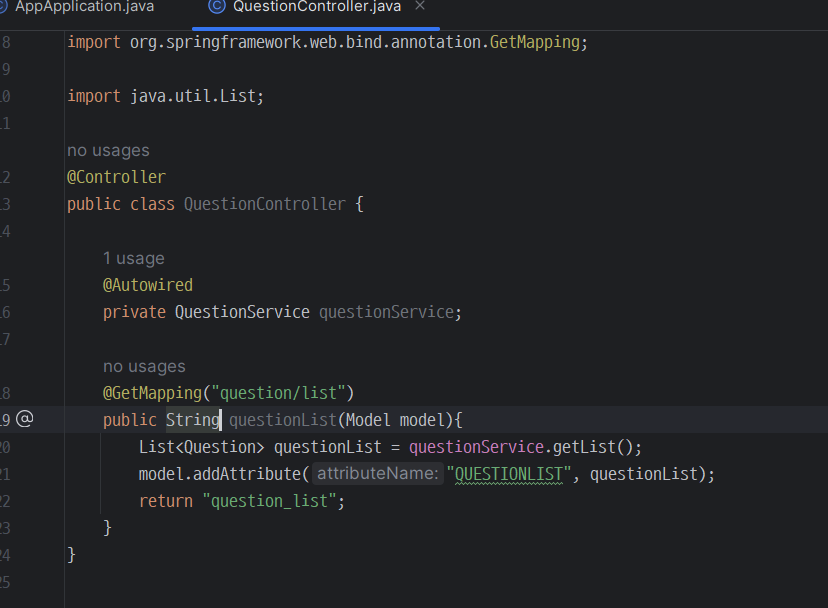
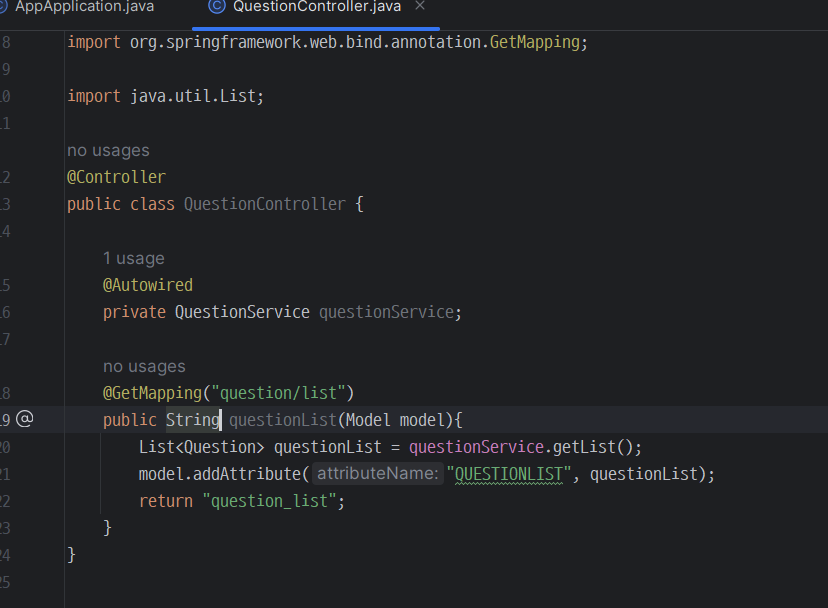
이전과 동일하게 매핑 시켜주고, 그때 보여줄 주소를 나타내자.
▶Service
더보기
package com.mycom2.app.question.controller;
import com.mycom2.app.question.service.QuestionService;
import com.mycom2.app.question.entity.Question;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import java.util.List;
@Controller
public class QuestionController {
@Autowired
private QuestionService questionService;
@GetMapping("question/list")
public String questionList(Model model){
List<Question> questionList = questionService.getList();
model.addAttribute("QUESTIONLIST", questionList);
return "question_list";
}
}
service까지도 기존과의 차이가 없지만, repository에서 설정되지 않아도 쓸수 있는 일부 method들이 존재한다는걸 미리 알아두자.
▶Repository (★)
더보기
package com.mycom2.app.question.repository;
import com.mycom2.app.question.entity.Question;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.jpa.repository.JpaRepository;
import java.util.List;
//Repository는 Entity에 의해 생성된 db에 접속해 작업하는 여러메서드들로 구성된 interface이다.
//JpaRepository 인터페이스를 상속하고있다.
//<Repository의 대상이 되는 Entitiy, Entity의 PK 타입>
public interface QuestionRepository extends JpaRepository<Question,Integer> {
Question findBySubject(String subject);
Question findBySubjectAndContent(String subject, String content);
List<Question> findBySubjectLike(String subject);
}
여기에서 결정적으로 큰 차이가 생긴다. 알아야 할 차이점으로는
1. Repository는 JpaRepositroy를 extends하며, 그 타입은 <PK, PK의타입>으로 적힌 것이다.
2. interface이기때문에 override될 method들을 문법을 지켜 만들어 주면 자동으로 DB와의 연동을 통해 return을 해주는 method 가 생성된다.
※사용문법
● 조회 (Retrieve) 단일 엔티티 조회: findById({id}), getById({id}) 모든 엔티티 조회: findAll() 특정 조건으로 엔티티 조회: findBy{Property}({property}) 특정 조건으로 엔티티 리스트 조회: findAllBy{Property}({property}) ●카운트 (Count) 엔티티 수 카운트: count() 특정 조건의 엔티티 수 카운트: countBy{Property}({property}) ● 추가 (Insert) 엔티티 추가: save({entity}), saveAndFlush({entity}) 여러 엔티티 추가: saveAll({entities}) ● 수정 (Update) 엔티티 수정: save({entity}), saveAndFlush({entity}) ● 삭제 (Delete) 엔티티 삭제: delete({entity}), deleteById({id}) 특정 조건의 엔티티 삭제: deleteBy{Property}({property}) 모든 엔티티 삭제: deleteAll() ● 쿼리 (Query) JPQL 쿼리 실행: @Query("JPQL query") 네이티브 SQL 쿼리 실행: @Query(value = "SQL query", nativeQuery = true) ● 정렬 (Sorting) 정렬하여 엔티티 조회: findAllByOrderBy{Property}Asc(), findAllByOrderBy{Property}Desc() ● 페이징 (Paging) 페이징 처리하여 엔티티 조회: findAll(Pageable pageable) ● 조인 (Join) 연관 관계를 사용한 엔티티 조회: findBy{RelatedEntity_Property}({relatedEntity}) 조인을 이용한 커스텀 쿼리 실행: @Query("SELECT ... FROM Entity e JOIN e.relatedEntity re WHERE ...") |
중괄호로 둘러싸인 부분은 Id나 property가 아니라 Column명으로 보면 되겠다.
모든 레코드, 단일 레코드, 특정레코드를 가져올 수 있다.
2. View
더보기
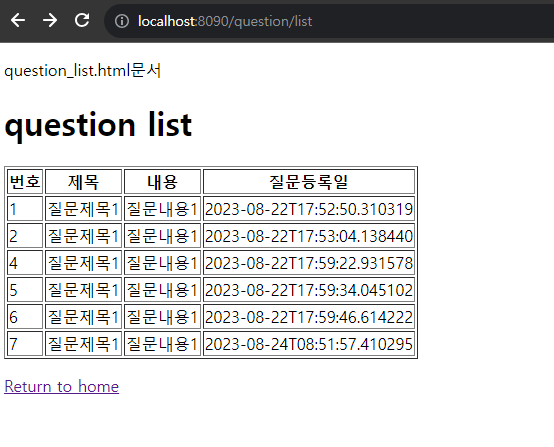
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>question_list</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" type="text/css"
href="/css/style.css" th:href="@{/css/style.css}" />
</head>
<body>
<p>question_list.html문서</p>
<h1>question list</h1>
<table border="1">
<tr>
<th>번호</th>
<th>제목</th>
<th>내용</th>
<th>질문등록일</th>
</tr>
<tr th:each="question : ${QUESTIONLIST}">
<td th:text="${question.id}">번호</td>
<td th:text="${question.subject}">제목</td>
<td th:text="${question.content}">내용</td>
<td th:text="${question.datetime}">날자</td>
</tr>
</table>
<p>
<a href="/" th:href="@{/}">Return to home</a>
</p>
</body>
</html>
이렇게 model에서 정한 어트리뷰트, 그리고 Controller가 지정한 위치에 정확한 html 파일이 존재한다면
thymeleaf 양식을 이용해서 틀을 만들어주자.
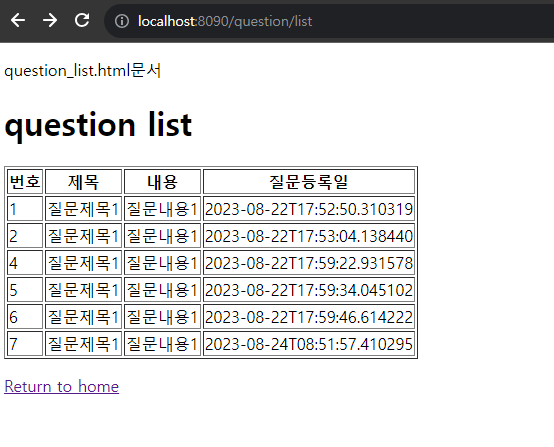
잘 설정해주었다면 이렇게 나올 것이다.
'Web > SpringBoot국비지원 수업 정리' 카테고리의 다른 글
<SpringBoot 국비지원 수업> H2 Database (0) | 2023.08.22 |
---|---|
<SpringBoot 국비지원 수업> Intelli-J 환경 사용하기 (0) | 2023.08.22 |
<SpringBoot 국비지원 수업> Thymeleaf (0) | 2023.08.21 |
<SpringBoot 국비지원 수업> 스프링에서 jsp를 사용하기 (0) | 2023.08.21 |
<SpringBoot 국비지원 수업> SpringBoot에서 Web 화면 생성 (0) | 2023.08.21 |